최근 회사에서 계좌확인 API와 카드사용내역 API를 연동해서 지출 내역을 한 번에 확인할 수 있는 기능을 추가하는 프로젝트를 하게 되었다. 원래는 은행별로 로그인하면서 확인해야 했었기 때문에 경영팀에서 조금 귀찮고 번거로웠다면서 올해가 가기 전에 개편을 하자고 했다. 그래서 관련 API를 제공하는 업체를 찾아보니 두 곳이 나왔는데 견적서도 받아보고 개발자센터에 설명도 잘 되어있나 확인도 해보고 난 후 가장 합리적인 바로빌을 선택하게 되었다.
[바로빌]
바로빌은 표준전자인증을 받아 전자(세금) 계산서 관련한 API를 제공하는 업체이다. 사실 나도 검색하다 알게 된 회사인데 제공하는 API도 많고, 사용자가 20만 정도가 된다고 한다. 또 홈택스 업데이트나 변경 사항 발생 시 바로빌 개발팀이 실시간으로 모니터링하고 바로 반영한다고 하니 가슴 졸이며 안 되는 이유를 내가 찾지 않아도 돼서 좋은 것 같다.. 핫...
바로빌 개발자센터 | 비즈니스 데이터 API 연동, 전자세금계산서 구축
솔루션/사이트/ERP에 세금계산서, 메시징, 스크래핑 등 API 연동서비스를 연결하여 기업에 필요한 비즈니스 데이터를 제공하는 API 전문기업
dev.barobill.co.kr
그리고 바로빌의 가장 좋은 점은 개발자 센터와 블로그가 너무 잘 되어 있는 것이다. 깔끔한 정리로 어떻게 코드를 구현해야 하는가 설명을 자세히 써 놓아서 너무 좋다... 심지어 샘플 코드도 자세해서 참고하기 아주 좋으니 주니어에게는 강 같은 글이 된다... 진짜루
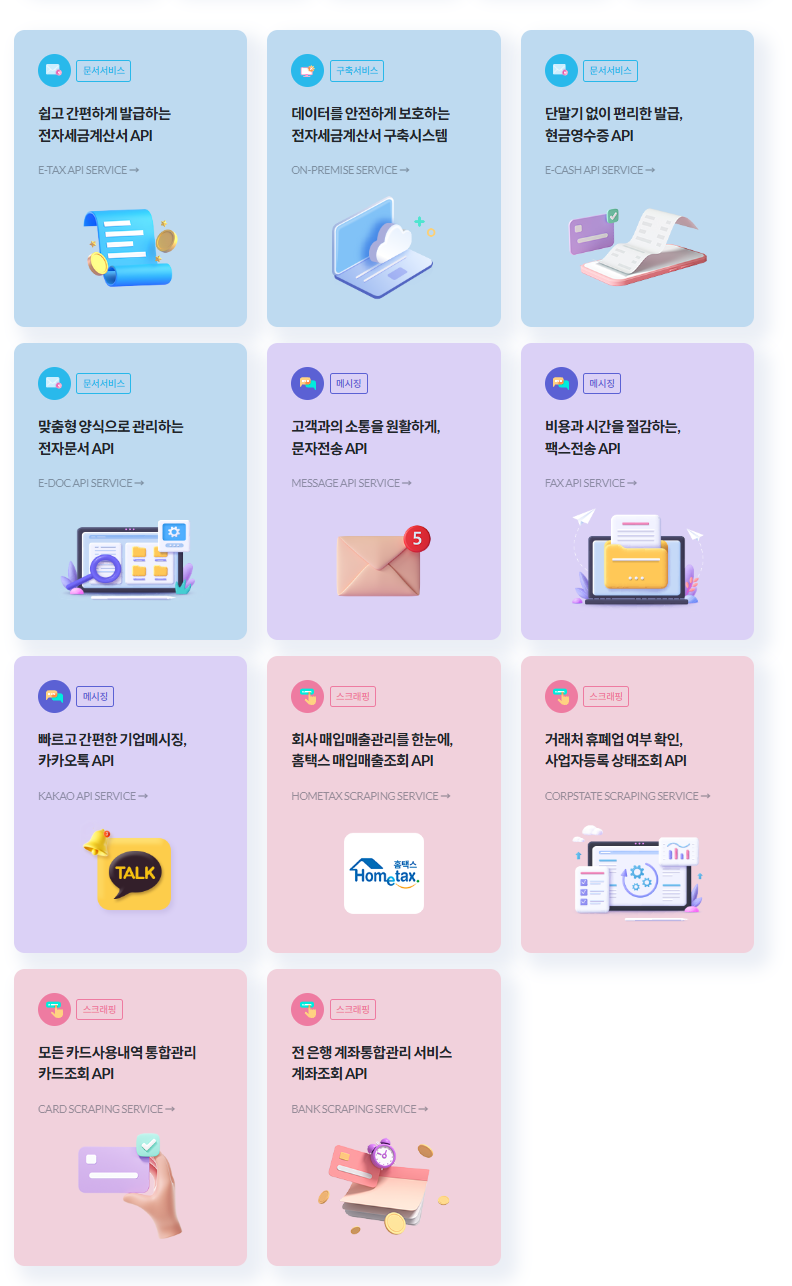
[계좌 확인 API - 기초 세팅]
바로빌의 계좌 확인 API는 은행 별로 로그인 할 필요 없이 바로빌 데이터 스크래핑 기술로 내역을 가져올 수 있다. 하지만 그전에 은행 별로 빠른 조회 서비스에 계좌가 등록되어 있어야 한다. 네이버나 구글에 은행 빠른 조회 혹은 간편 조회라고 치면 바로 나오니까 어렵지 않게 설정할 수 있다.
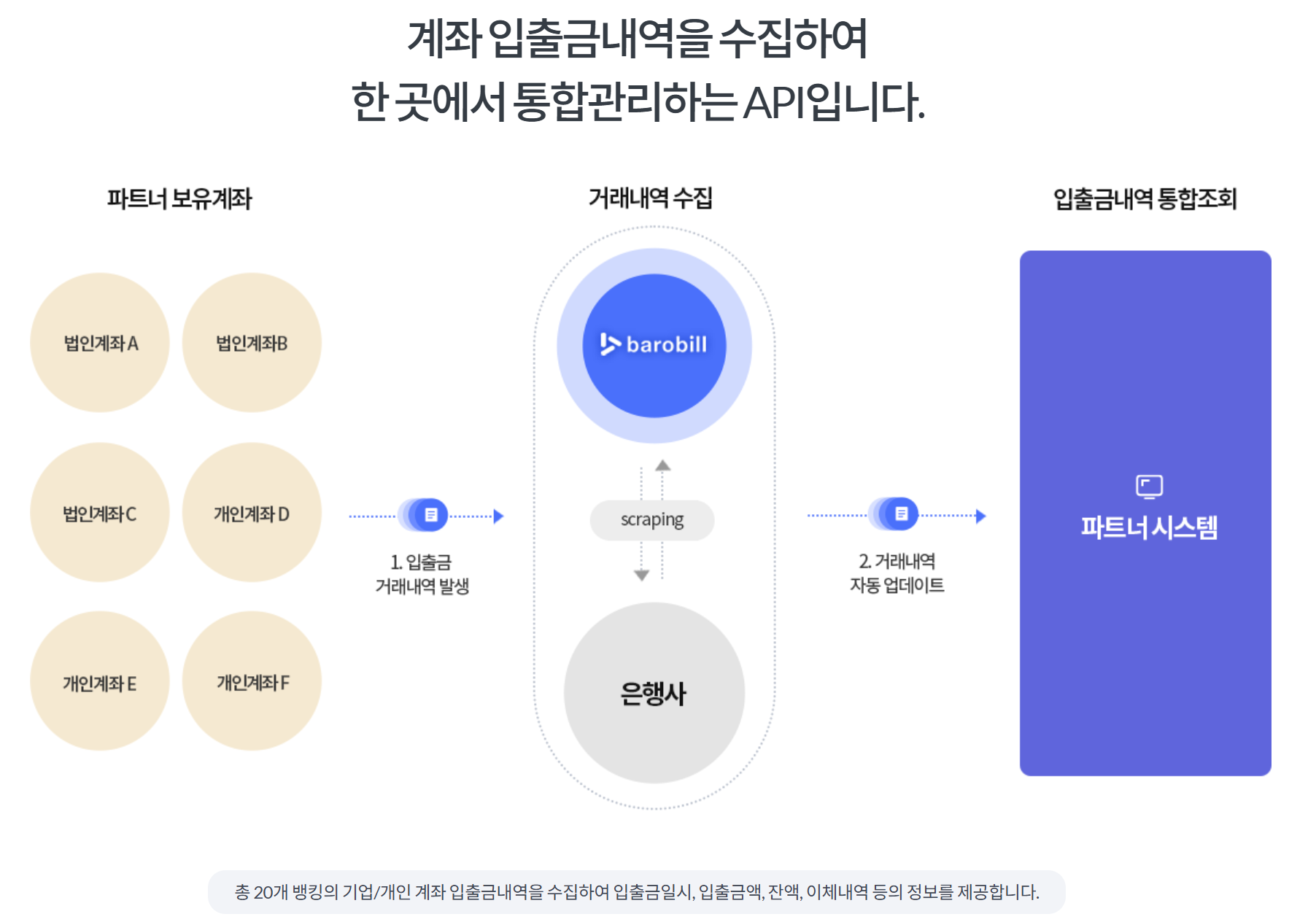
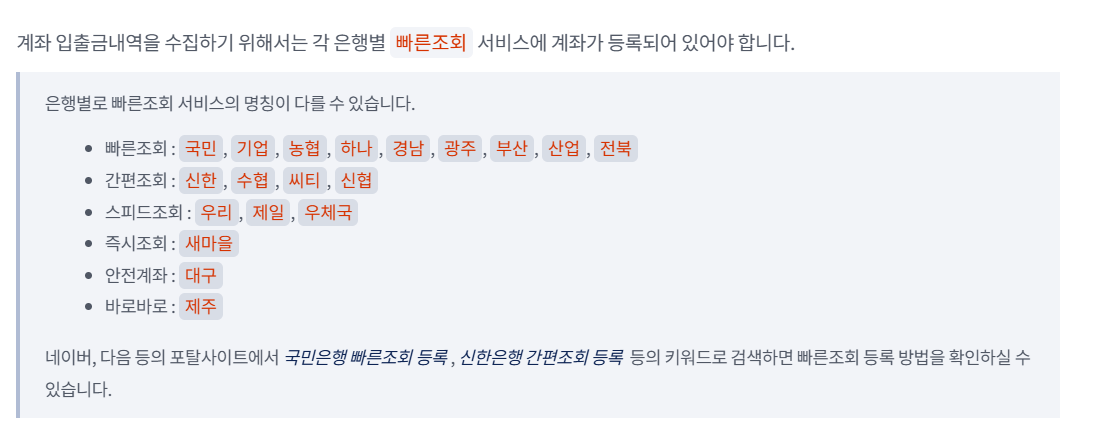
[계좌 확인 API - 계좌 등록]
아래 처럼 계좌를 등록하는 클래스를 만들어서 계좌확인 API에 사용할 계좌들을 등록해 준다.
public class BankAccountRegi{
public static void main(String[] args) {
BarobillApiService barobillApiService = new BarobillApiService();
// 연동 인증키, 사업자 번호, 계좌 정보 입력
String certKey = "yourCertKey"; // 연동 인증키 입력
String corpNum = "1234567890"; // 사업자 번호 입력
String collectCycle = "30"; // 수집 주기 (예: 30분마다 수집)
String bank = "004"; // 은행 코드 (예: KB국민은행 코드 004)
String bankAccountType = "01"; // 계좌 유형 (01: 입출금)
String bankAccountNum = "1234567890123"; // 계좌 번호 (하이픈 제외)
// 호출 결과 저장
int result = barobillApiService.bankAccount.registBankAccount(certKey, corpNum, collectCycle, bank, bankAccountType, bankAccountNum);
// 결과 출력
if (result < 0) { // 호출 실패
System.out.println("API 호출 실패. 오류 코드: " + result);
} else { // 호출 성공
System.out.println("API 호출 성공. 결과 코드: " + result);
}
}
}
[계좌 확인 API - 계좌 목록 조회]
계좌를 등록 해보았으면 계좌를 조회를 해보는 것이 인지상정.. 객체를 생성해 주고 계좌조회 코드를 실행해 준다.
public class BankAccountList {
public static void main(String[] args) {
// 바로빌 서비스 객체 생성
BarobillApiService barobillApiService = new BarobillApiService();
// 계좌 조회 실행
getBankAccountEx(barobillApiService);
}
public static void getBankAccountEx(BarobillApiService barobillApiService) {
// 필수 입력 정보
String certKey = "YOUR_CERT_KEY"; // 연동 인증키
String corpNum = "1234567890"; // 사업자 번호
int availOnly = 1; // 사용 가능한 계좌만 조회
try {
// API 호출
List<BankAccount> bankAccounts = barobillApiService.bankAccount.getBankAccountEx(certKey, corpNum, availOnly).getBankAccount();
// 조회 결과 검증
if (bankAccounts == null || bankAccounts.isEmpty()) {
System.out.println("조회된 계좌가 없습니다.");
return;
}
// 결과 출력
if (bankAccounts.size() == 1 && isErrorAccount(bankAccounts.get(0).getBankAccountNum())) {
System.out.println("오류 코드 계좌: " + bankAccounts.get(0).getBankAccountNum());
} else {
System.out.println("조회된 계좌 목록:");
for (BankAccount account : bankAccounts) {
System.out.println("계좌번호: " + account.getBankAccountNum());
System.out.println("은행명: " + account.getBankName());
System.out.println("계좌 유형: " + account.getBankAccountType());
System.out.println("---------------------------------");
}
}
} catch (Exception e) {
System.err.println("API 호출 중 오류 발생: " + e.getMessage());
e.printStackTrace();
}
}
}
}
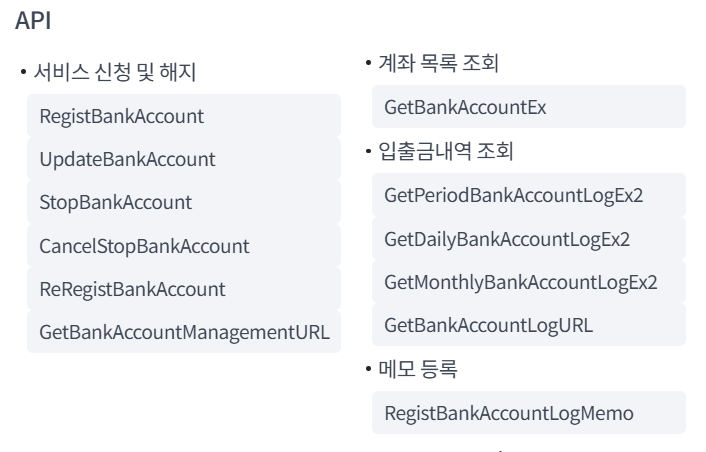
이거 외에도 계좌확인API에는 옆에 보이는 것과 같이 다양한 API 서비스를 제공하고 있으니 원하는 걸 사용하여 효율적인 코드를 작성하면 좋을 것 같다!
[카드사용내역 API - 기초세팅]
이번에는 카드사용 내역 API를 구현해보려고 한다. 사실 회사에서는 계좌확인 API 보다 중요한 것이 법인카드의 사용 내역을 수집하는 것이다. 아무래도 어디에 사용했는지 궁금하시겠지... 이것도 계좌확인 API처럼 먼저 세팅해야 할 것이 있으니 링크를 통해 확인 후 세팅해 주면 된다.
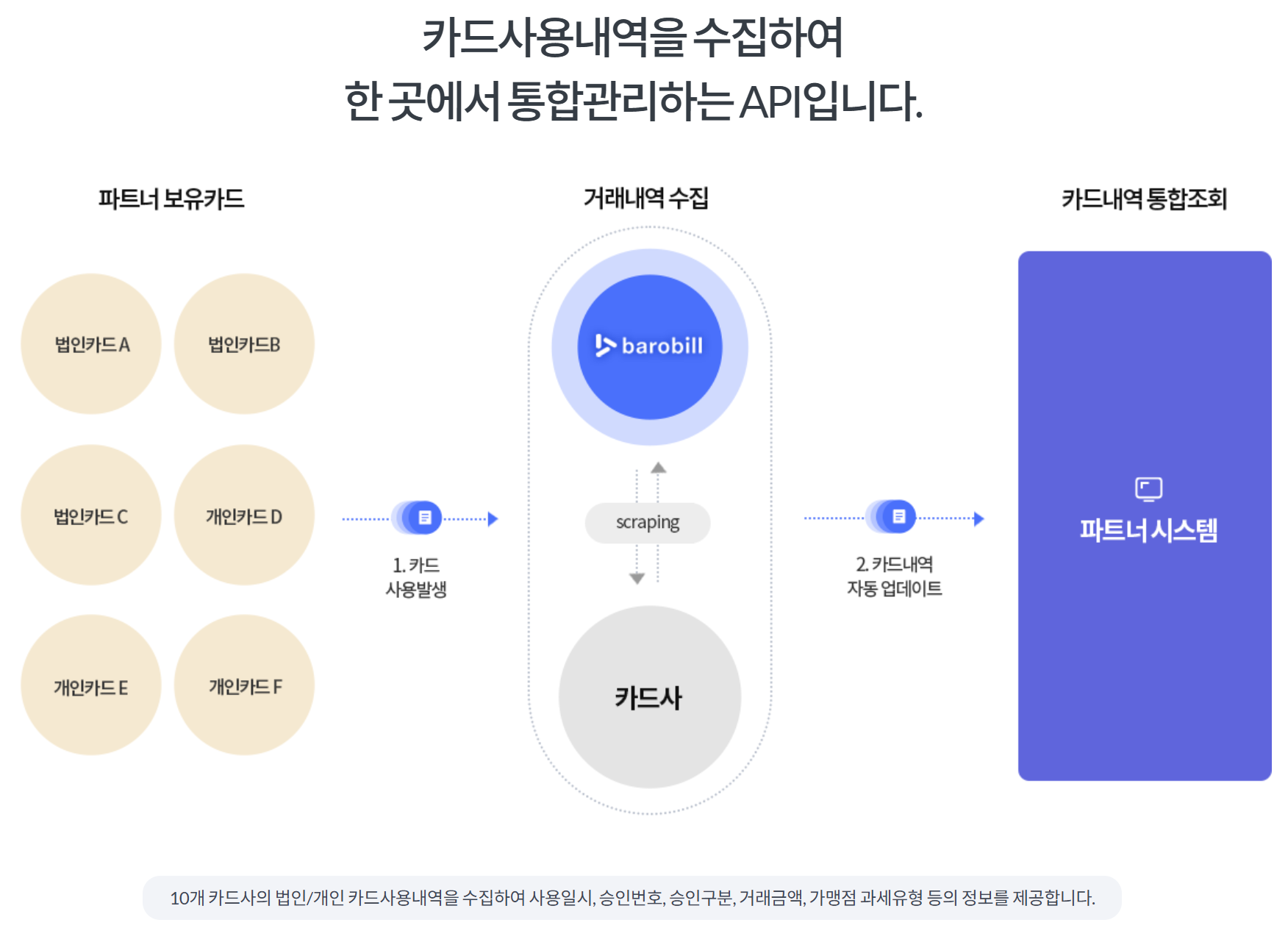
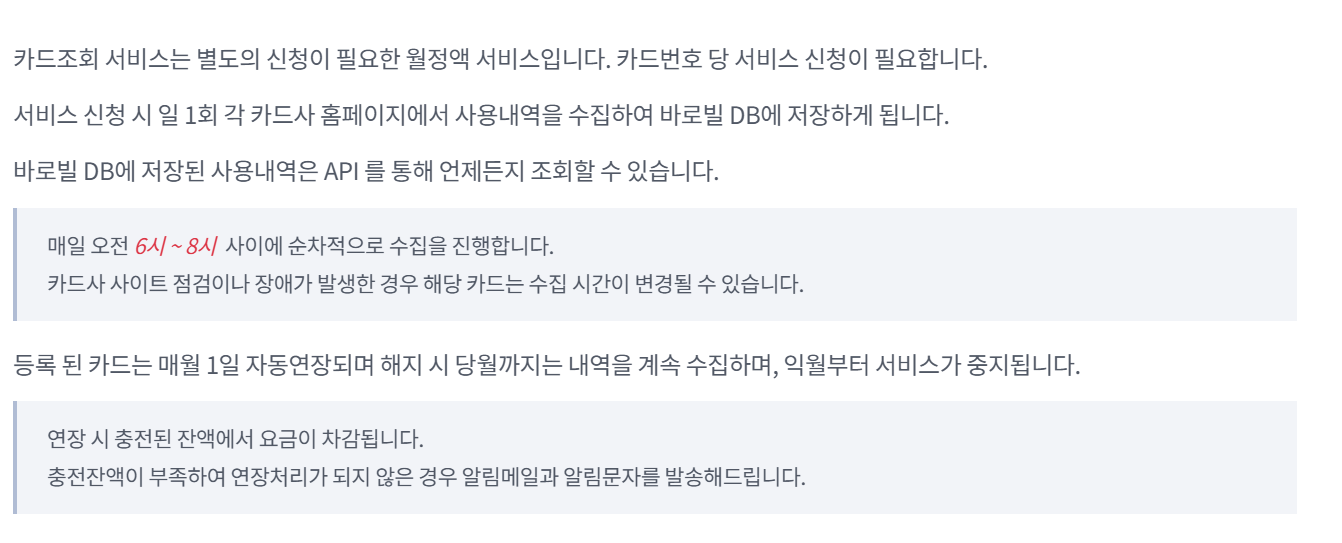
스크래핑 API 레퍼런스 - 카드조회 API | 바로빌 개발자센터
카드 등록 신청, 카드목록 조회, 카드 사용내역 조회 등 바로빌에서 제공하는 카드조회 API를 사용하는데 필요한 규격과 가이드를 제공합니다.
dev.barobill.co.kr
[카드사용내역 API - 카드 등록하기]
public class CardRegistrationTest {
public static void main(String[] args) {
// 바롬빌 API 서비스 객체 생성
BarobillApiService barobillApiService = new BarobillApiService();
// 카드 등록 메서드 실행
registCard(barobillApiService);
}
public static void registCard(BarobillApiService barobillApiService) {
// 사용자 입력을 위한 Scanner
Scanner scanner = new Scanner(System.in);
try {
// 입력 정보 수집
System.out.print("연동 인증키: ");
String certKey = scanner.nextLine();
System.out.print("사업자 번호: ");
String corpNum = scanner.nextLine();
System.out.print("카드사 코드: ");
String cardCompany = scanner.nextLine();
System.out.print("카드 유형 (법인/개인): ");
String cardType = scanner.nextLine();
System.out.print("카드 번호: ");
String cardNum = scanner.nextLine();
System.out.print("카드사 홈페이지 아이디: ");
String webId = scanner.nextLine();
System.out.print("카드사 홈페이지 비밀번호: ");
String webPwd = scanner.nextLine();
// API 호출
int result = barobillApiService.card.registCard(certKey, corpNum, cardCompany, cardType, cardNum, webId, webPwd, alias, usage);
// 결과 처리
if (result < 0) {
System.out.println("카드 등록 실패. 오류 코드: " + result);
} else {
System.out.println("카드 등록 성공. 결과 코드: " + result);
}
} catch (Exception e) {
System.err.println("카드 등록 중 오류 발생: " + e.getMessage());
e.printStackTrace();
} finally {
scanner.close();
}
}
}
[카드사용내역 API - 카드 목록조회]
이번에는 Card, CardResponse, CardService 클래스를 분리하여 데이터 모델과 로직이 구분 되게 코드를 짜보았다.
Card 정보 클래스
// 카드 정보 객체
class Card {
private String cardNum;
private String cardCompany;
private String cardType;
public Card(String cardNum, String cardCompany, String cardType) {
this.cardNum = cardNum;
this.cardCompany = cardCompany;
this.cardType = cardType;
}
public String getCardNum() {
return cardNum;
}
public String getCardCompany() {
return cardCompany;
}
public String getCardType() {
return cardType;
}
}
응답을 받을 객체 생성
class CardResponse {
private List<Card> card;
public List<Card> getCard() {
return card;
}
public void setCard(List<Card> card) {
this.card = card;
}
}
서비스 호출 클래스 생성
public class CardListService {
public static void main(String[] args) {
BarobillApiService barobillApiService = new BarobillApiService();
getCardEx(barobillApiService);
}
public static void getCardEx(BarobillApiService barobillApiService) {
// 입력값 초기화
String certKey = "YOUR_CERT_KEY"; // 연동 인증키 입력
String corpNum = "1234567890"; // 사업자 번호 입력
int availOnly = 1; // 0 : 모든 카드 1 : 사용 가능 카드만 조회
try {
// API 호출
List<Card> cardList = barobillApiService.card.getCardEx(certKey, corpNum, availOnly).getCard();
// 결과 처리
if (cardList == null || cardList.isEmpty()) {
System.out.println("조회된 카드가 없습니다.");
return;
}
if (isErrorCardList(cardList)) {
System.out.println("오류 코드 카드: " + cardList.get(0).getCardNum());
} else {
System.out.println("조회된 카드 목록:");
for (Card card : cardList) {
System.out.println("카드 번호: " + card.getCardNum());
System.out.println("카드사: " + card.getCardCompany());
System.out.println("---------------------------------");
}
}
} catch (Exception e) {
System.err.println("카드 조회 중 오류 발생: " + e.getMessage());
e.printStackTrace();
}
}
// 오류 카드 목록 확인 (예: -12345 패턴)
private static boolean isErrorCardList(List<Card> cardList) {
return cardList.size() == 1 && Pattern.compile("^-[0-9]{5}$").matcher(cardList.get(0).getCardNum()).matches();
}
}
[카드사용내역 API - 카드 사용내역 조회]
바로빌에서 제공하는 카드 사용 내역 조회 API 는 크게 4가지로 나뉜다.
- 원하는 날짜 (단, 200일 사이) 를 지정해서 하는 방법
- 1일씩 조회 하는 것
- 1개월분씩 조회하는 것
- 카드 사용 내역을 조회할 수 있는 URL을 반환
나는 조회 후 결과를 반환하는 세 가지 방법을 구현해보겠다.
먼저 리턴 값을 받을 PagedCardLogEx3 클래스를 생성해 준다.
import java.util.List;
public class PagedCardLogEx3 {
private int currentPage; // 현재 페이지
private int countPerPage; // 페이지 당 건수
private int maxPageNum; // 최대 페이지 수
private int maxIndex; // 전체 건수
private CardLogList cardLogList; // 카드 사용내역 목록
// 기본 생성자
public PagedCardLogEx3() {}
// 필드 전체 초기화 생성자
public PagedCardLogEx3(int currentPage, int countPerPage, int maxPageNum, int maxIndex, CardLogList cardLogList) {
this.currentPage = currentPage;
this.countPerPage = countPerPage;
this.maxPageNum = maxPageNum;
this.maxIndex = maxIndex;
this.cardLogList = cardLogList;
}
// Getter와 Setter
public int getCurrentPage() {
return currentPage;
}
public void setCurrentPage(int currentPage) {
this.currentPage = currentPage;
}
public int getCountPerPage() {
return countPerPage;
}
public void setCountPerPage(int countPerPage) {
this.countPerPage = countPerPage;
}
public int getMaxPageNum() {
return maxPageNum;
}
public void setMaxPageNum(int maxPageNum) {
this.maxPageNum = maxPageNum;
}
public int getMaxIndex() {
return maxIndex;
}
public void setMaxIndex(int maxIndex) {
this.maxIndex = maxIndex;
}
public CardLogList getCardLogList() {
return cardLogList;
}
public void setCardLogList(CardLogList cardLogList) {
this.cardLogList = cardLogList;
}
// 내부 클래스: CardLogList
public static class CardLogList {
private List<CardLogEx3> cardLogEx3;
public CardLogList() {}
public CardLogList(List<CardLogEx3> cardLogEx3) {
this.cardLogEx3 = cardLogEx3;
}
public List<CardLogEx3> getCardLogEx3() {
return cardLogEx3;
}
public void setCardLogEx3(List<CardLogEx3> cardLogEx3) {
this.cardLogEx3 = cardLogEx3;
}
}
}
카드사용내역 API 호출이 비슷하니 공통으로 사용할 서비스 클래스도 만들어 준다.
import java.util.List;
public class CardUseList {
private final BarobillApiService barobillApiService = new BarobillApiService();
// 월 카드사용내역 API 요청
public PagedCardLogEx3 getMonthlyCardLogEx3(String certKey, String corpNum, String id, String cardNum, String baseMonth, int countPerPage, int currentPage, int orderDirection) {
return fetchCardLog("Monthly", certKey, corpNum, id, cardNum, baseMonth, null, countPerPage, currentPage, orderDirection);
}
// 일 카드사용내역 API 요청
public PagedCardLogEx3 getDailyCardLogEx3(String certKey, String corpNum, String id, String cardNum, String baseDate, int countPerPage, int currentPage, int orderDirection) {
return fetchCardLog("Daily", certKey, corpNum, id, cardNum, baseDate, null, countPerPage, currentPage, orderDirection);
}
// 기간 카드사용내역 API 요청
public PagedCardLogEx3 getPeriodCardLogEx3(String certKey, String corpNum, String id, String cardNum, String startDate, String endDate, int countPerPage, int currentPage, int orderDirection) {
return fetchCardLog("Period", certKey, corpNum, id, cardNum, startDate, endDate, countPerPage, currentPage, orderDirection);
}
private PagedCardLogEx3 fetchCardLog(String type, String certKey, String corpNum, String id, String cardNum, String date1, String date2, int countPerPage, int currentPage, int orderDirection) {
PagedCardLogEx3 result = null;
switch (type) {
case "Monthly":
result = barobillApiService.card.getMonthlyCardLogEx3(certKey, corpNum, id, cardNum, date1, countPerPage, currentPage, orderDirection);
break;
case "Daily":
result = barobillApiService.card.getDailyCardLogEx3(certKey, corpNum, id, cardNum, date1, countPerPage, currentPage, orderDirection);
break;
case "Period":
result = barobillApiService.card.getPeriodCardLogEx3(certKey, corpNum, id, cardNum, date1, date2, countPerPage, currentPage, orderDirection);
break;
}
printCardLogResult(result);
return result;
}
private void printCardLogResult(PagedCardLogEx3 result) {
if (result.getCurrentPage() < 0) { // 호출 실패
System.out.println("호출 실패: " + result.getCurrentPage());
} else { // 호출 성공
System.out.printf("페이지: %d / 페이지당 항목 수: %d / 최대 페이지: %d / 최대 항목 수: %d%n",
result.getCurrentPage(), result.getCountPerPage(), result.getMaxPageNum(), result.getMaxIndex());
List<CardLogEx3> logs = result.getCardLogList().getCardLogEx3();
for (CardLogEx3 log : logs) {
System.out.println("카드 번호: " + log.getCardNum());
}
}
}
}
이걸 이제 메인에서 사용해주면 원하는 카드사용내역을 조회할 수 있다.
[마무리]
테스트 코드를 조금 변경 해서 작성해 보았는데 바로빌은 제공하는 정보가 너무 깔끔하고 문서화가 잘 되어 있어서 사용하기 편리하다. 또 원하는 API 서비스만 선택하여 개발한 후 사용한 만큼만 지불하는 시스템이라 가격적인 측면에서도 너무 매력적인 것 같다.
조금 더 살펴보길 원한다면 아래의 링크로 가서 둘러보시는걸 추천드립니당
스크래핑 API 레퍼런스 - 카드조회 API | 바로빌 개발자센터
카드 등록 신청, 카드목록 조회, 카드 사용내역 조회 등 바로빌에서 제공하는 카드조회 API를 사용하는데 필요한 규격과 가이드를 제공합니다.
dev.barobill.co.kr
해당 게시글은 업체로부터 소정의 원고료를 받아 작성되었습니다
'JAVA' 카테고리의 다른 글
[자료구조] Array 와 List 의 차이 (배열과 리스트의 차이) (0) | 2024.11.27 |
---|---|
BufferedReader 와 BufferedWriter (0) | 2024.11.23 |
Java int배열의 오름차순과 내림차순 (0) | 2024.11.21 |
Java int 와 Integer 의 차이 (0) | 2024.11.20 |
Java 문자열 뒤집기 - reverse() (0) | 2024.11.19 |
최근 회사에서 계좌확인 API와 카드사용내역 API를 연동해서 지출 내역을 한 번에 확인할 수 있는 기능을 추가하는 프로젝트를 하게 되었다. 원래는 은행별로 로그인하면서 확인해야 했었기 때문에 경영팀에서 조금 귀찮고 번거로웠다면서 올해가 가기 전에 개편을 하자고 했다. 그래서 관련 API를 제공하는 업체를 찾아보니 두 곳이 나왔는데 견적서도 받아보고 개발자센터에 설명도 잘 되어있나 확인도 해보고 난 후 가장 합리적인 바로빌을 선택하게 되었다.
[바로빌]
바로빌은 표준전자인증을 받아 전자(세금) 계산서 관련한 API를 제공하는 업체이다. 사실 나도 검색하다 알게 된 회사인데 제공하는 API도 많고, 사용자가 20만 정도가 된다고 한다. 또 홈택스 업데이트나 변경 사항 발생 시 바로빌 개발팀이 실시간으로 모니터링하고 바로 반영한다고 하니 가슴 졸이며 안 되는 이유를 내가 찾지 않아도 돼서 좋은 것 같다.. 핫...
바로빌 개발자센터 | 비즈니스 데이터 API 연동, 전자세금계산서 구축
솔루션/사이트/ERP에 세금계산서, 메시징, 스크래핑 등 API 연동서비스를 연결하여 기업에 필요한 비즈니스 데이터를 제공하는 API 전문기업
dev.barobill.co.kr
그리고 바로빌의 가장 좋은 점은 개발자 센터와 블로그가 너무 잘 되어 있는 것이다. 깔끔한 정리로 어떻게 코드를 구현해야 하는가 설명을 자세히 써 놓아서 너무 좋다... 심지어 샘플 코드도 자세해서 참고하기 아주 좋으니 주니어에게는 강 같은 글이 된다... 진짜루
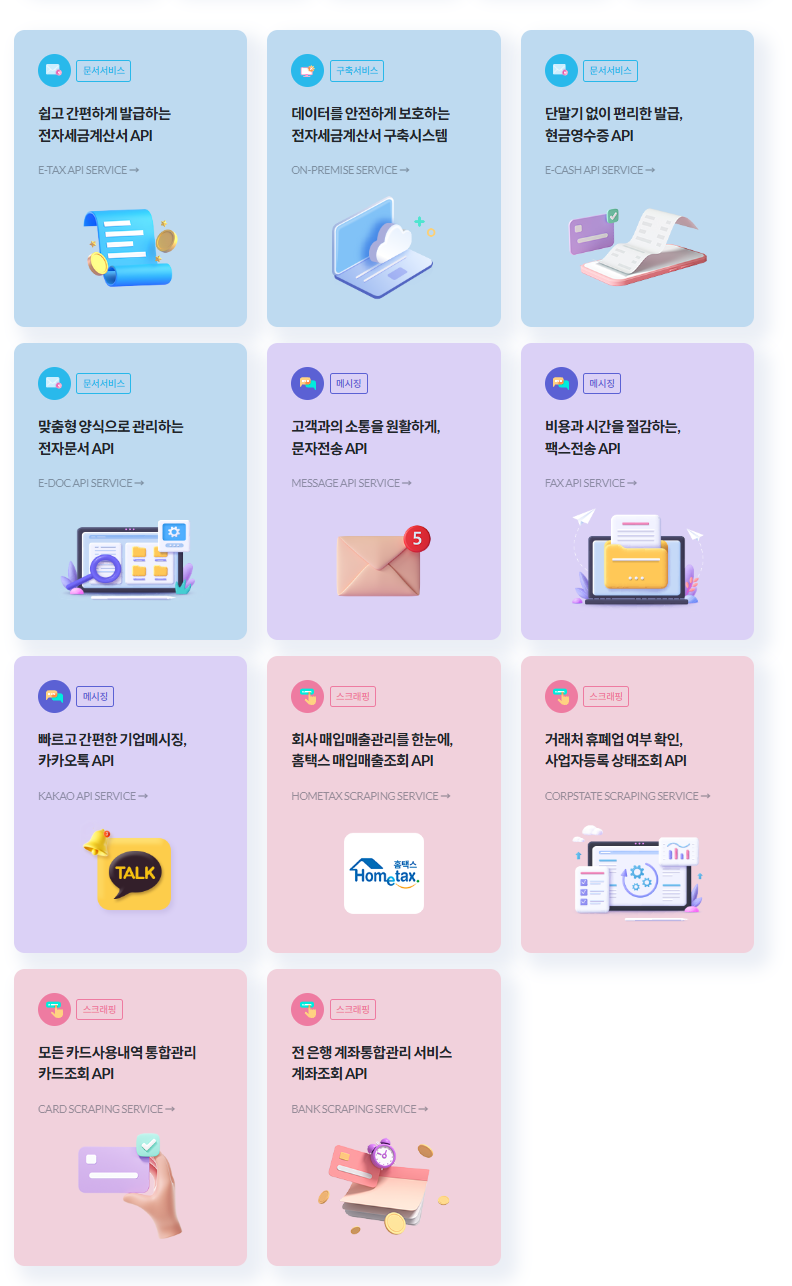
[계좌 확인 API - 기초 세팅]
바로빌의 계좌 확인 API는 은행 별로 로그인 할 필요 없이 바로빌 데이터 스크래핑 기술로 내역을 가져올 수 있다. 하지만 그전에 은행 별로 빠른 조회 서비스에 계좌가 등록되어 있어야 한다. 네이버나 구글에 은행 빠른 조회 혹은 간편 조회라고 치면 바로 나오니까 어렵지 않게 설정할 수 있다.
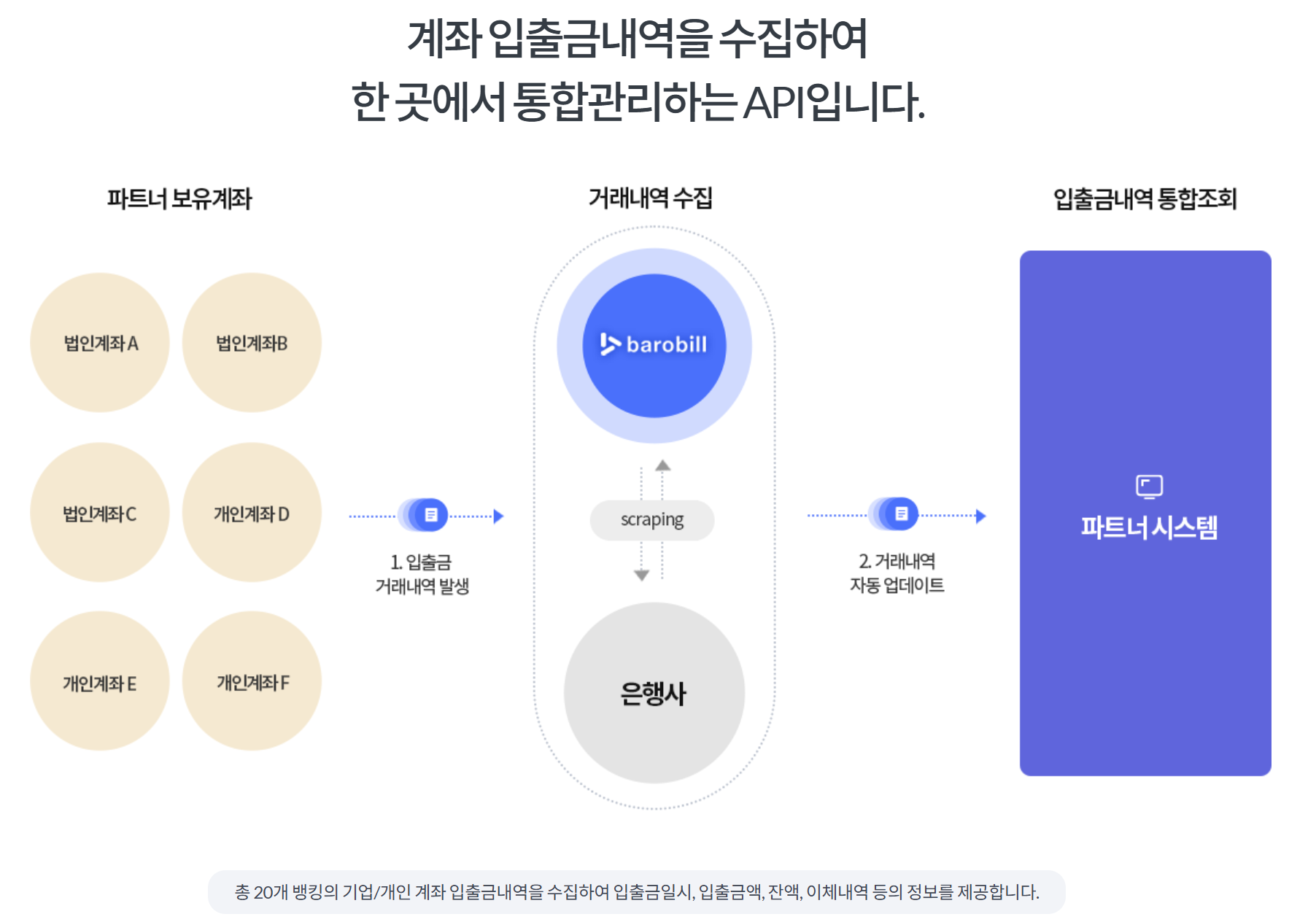
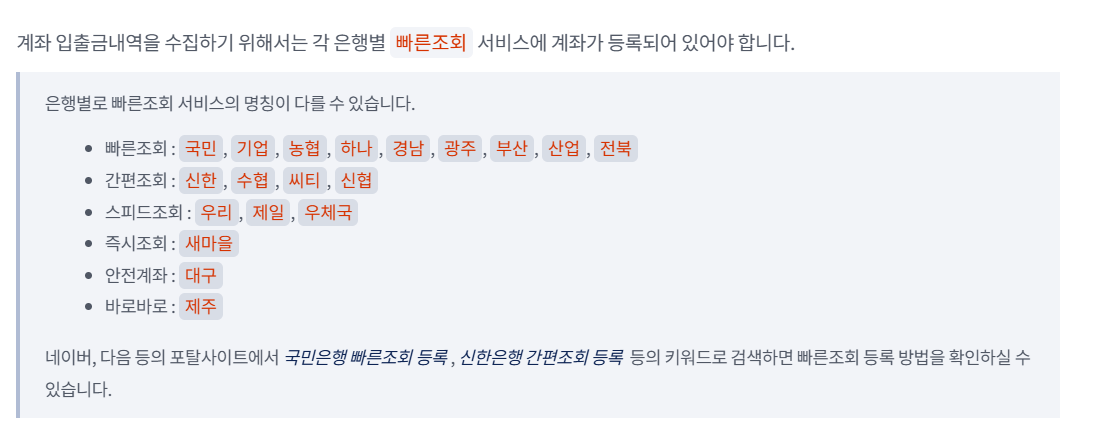
[계좌 확인 API - 계좌 등록]
아래 처럼 계좌를 등록하는 클래스를 만들어서 계좌확인 API에 사용할 계좌들을 등록해 준다.
public class BankAccountRegi{
public static void main(String[] args) {
BarobillApiService barobillApiService = new BarobillApiService();
// 연동 인증키, 사업자 번호, 계좌 정보 입력
String certKey = "yourCertKey"; // 연동 인증키 입력
String corpNum = "1234567890"; // 사업자 번호 입력
String collectCycle = "30"; // 수집 주기 (예: 30분마다 수집)
String bank = "004"; // 은행 코드 (예: KB국민은행 코드 004)
String bankAccountType = "01"; // 계좌 유형 (01: 입출금)
String bankAccountNum = "1234567890123"; // 계좌 번호 (하이픈 제외)
// 호출 결과 저장
int result = barobillApiService.bankAccount.registBankAccount(certKey, corpNum, collectCycle, bank, bankAccountType, bankAccountNum);
// 결과 출력
if (result < 0) { // 호출 실패
System.out.println("API 호출 실패. 오류 코드: " + result);
} else { // 호출 성공
System.out.println("API 호출 성공. 결과 코드: " + result);
}
}
}
[계좌 확인 API - 계좌 목록 조회]
계좌를 등록 해보았으면 계좌를 조회를 해보는 것이 인지상정.. 객체를 생성해 주고 계좌조회 코드를 실행해 준다.
public class BankAccountList {
public static void main(String[] args) {
// 바로빌 서비스 객체 생성
BarobillApiService barobillApiService = new BarobillApiService();
// 계좌 조회 실행
getBankAccountEx(barobillApiService);
}
public static void getBankAccountEx(BarobillApiService barobillApiService) {
// 필수 입력 정보
String certKey = "YOUR_CERT_KEY"; // 연동 인증키
String corpNum = "1234567890"; // 사업자 번호
int availOnly = 1; // 사용 가능한 계좌만 조회
try {
// API 호출
List<BankAccount> bankAccounts = barobillApiService.bankAccount.getBankAccountEx(certKey, corpNum, availOnly).getBankAccount();
// 조회 결과 검증
if (bankAccounts == null || bankAccounts.isEmpty()) {
System.out.println("조회된 계좌가 없습니다.");
return;
}
// 결과 출력
if (bankAccounts.size() == 1 && isErrorAccount(bankAccounts.get(0).getBankAccountNum())) {
System.out.println("오류 코드 계좌: " + bankAccounts.get(0).getBankAccountNum());
} else {
System.out.println("조회된 계좌 목록:");
for (BankAccount account : bankAccounts) {
System.out.println("계좌번호: " + account.getBankAccountNum());
System.out.println("은행명: " + account.getBankName());
System.out.println("계좌 유형: " + account.getBankAccountType());
System.out.println("---------------------------------");
}
}
} catch (Exception e) {
System.err.println("API 호출 중 오류 발생: " + e.getMessage());
e.printStackTrace();
}
}
}
}
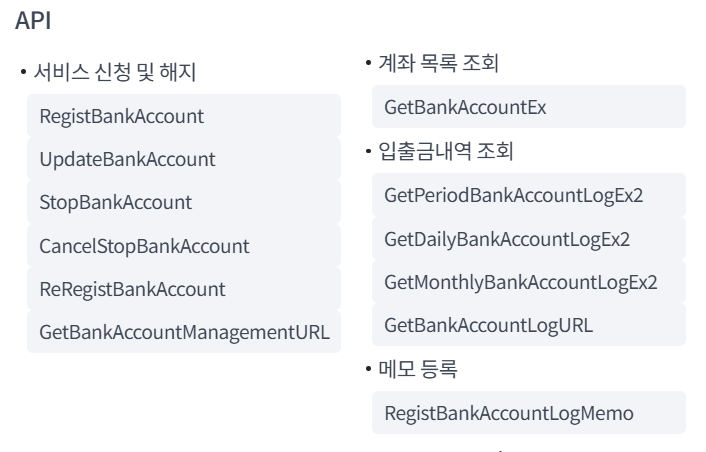
이거 외에도 계좌확인API에는 옆에 보이는 것과 같이 다양한 API 서비스를 제공하고 있으니 원하는 걸 사용하여 효율적인 코드를 작성하면 좋을 것 같다!
[카드사용내역 API - 기초세팅]
이번에는 카드사용 내역 API를 구현해보려고 한다. 사실 회사에서는 계좌확인 API 보다 중요한 것이 법인카드의 사용 내역을 수집하는 것이다. 아무래도 어디에 사용했는지 궁금하시겠지... 이것도 계좌확인 API처럼 먼저 세팅해야 할 것이 있으니 링크를 통해 확인 후 세팅해 주면 된다.
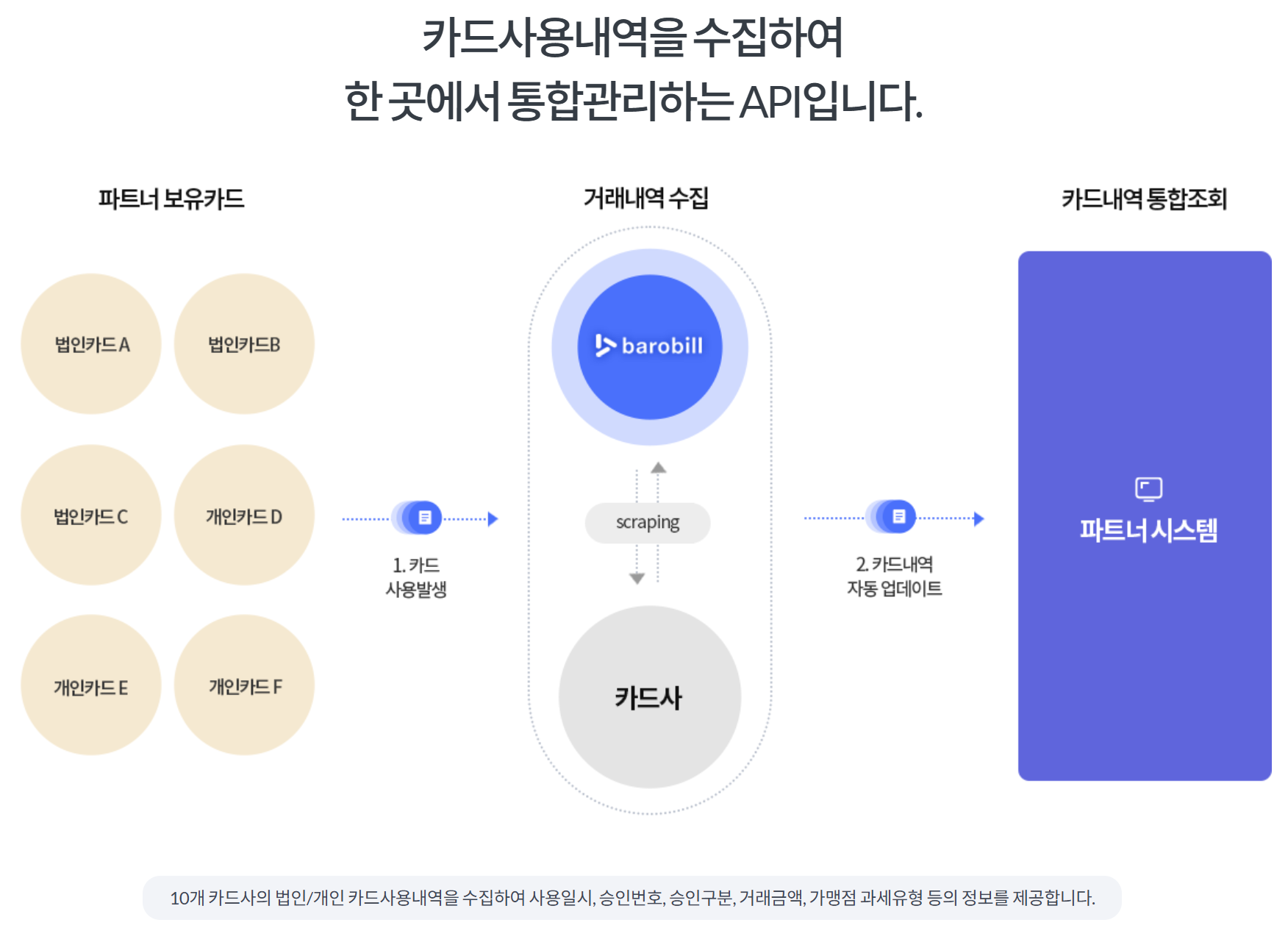
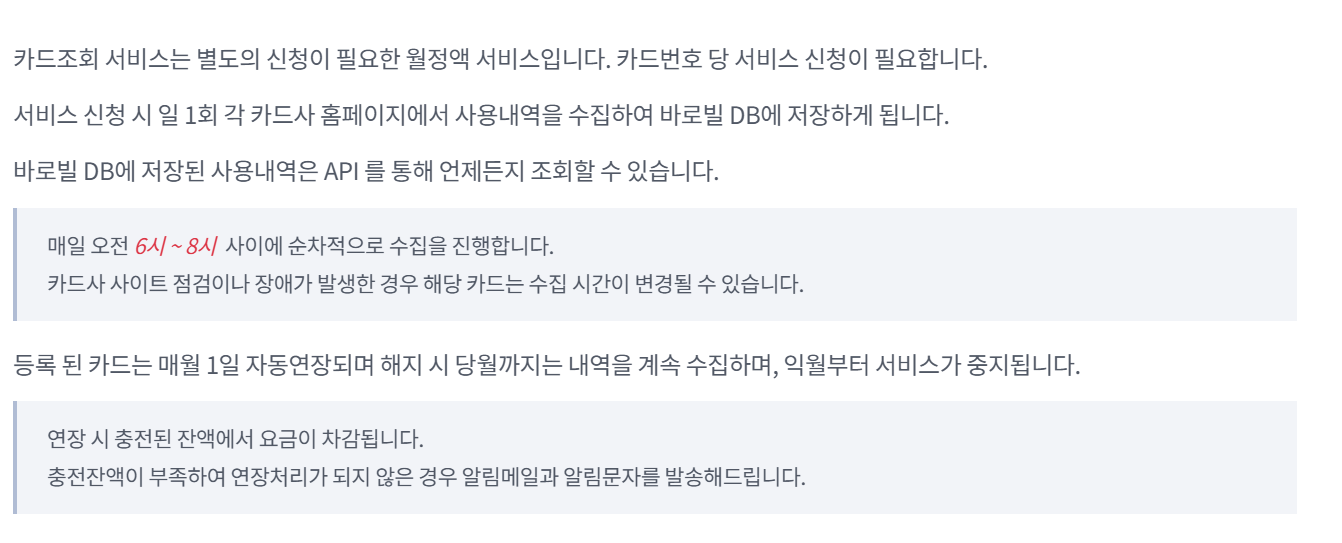
스크래핑 API 레퍼런스 - 카드조회 API | 바로빌 개발자센터
카드 등록 신청, 카드목록 조회, 카드 사용내역 조회 등 바로빌에서 제공하는 카드조회 API를 사용하는데 필요한 규격과 가이드를 제공합니다.
dev.barobill.co.kr
[카드사용내역 API - 카드 등록하기]
public class CardRegistrationTest {
public static void main(String[] args) {
// 바롬빌 API 서비스 객체 생성
BarobillApiService barobillApiService = new BarobillApiService();
// 카드 등록 메서드 실행
registCard(barobillApiService);
}
public static void registCard(BarobillApiService barobillApiService) {
// 사용자 입력을 위한 Scanner
Scanner scanner = new Scanner(System.in);
try {
// 입력 정보 수집
System.out.print("연동 인증키: ");
String certKey = scanner.nextLine();
System.out.print("사업자 번호: ");
String corpNum = scanner.nextLine();
System.out.print("카드사 코드: ");
String cardCompany = scanner.nextLine();
System.out.print("카드 유형 (법인/개인): ");
String cardType = scanner.nextLine();
System.out.print("카드 번호: ");
String cardNum = scanner.nextLine();
System.out.print("카드사 홈페이지 아이디: ");
String webId = scanner.nextLine();
System.out.print("카드사 홈페이지 비밀번호: ");
String webPwd = scanner.nextLine();
// API 호출
int result = barobillApiService.card.registCard(certKey, corpNum, cardCompany, cardType, cardNum, webId, webPwd, alias, usage);
// 결과 처리
if (result < 0) {
System.out.println("카드 등록 실패. 오류 코드: " + result);
} else {
System.out.println("카드 등록 성공. 결과 코드: " + result);
}
} catch (Exception e) {
System.err.println("카드 등록 중 오류 발생: " + e.getMessage());
e.printStackTrace();
} finally {
scanner.close();
}
}
}
[카드사용내역 API - 카드 목록조회]
이번에는 Card, CardResponse, CardService 클래스를 분리하여 데이터 모델과 로직이 구분 되게 코드를 짜보았다.
Card 정보 클래스
// 카드 정보 객체
class Card {
private String cardNum;
private String cardCompany;
private String cardType;
public Card(String cardNum, String cardCompany, String cardType) {
this.cardNum = cardNum;
this.cardCompany = cardCompany;
this.cardType = cardType;
}
public String getCardNum() {
return cardNum;
}
public String getCardCompany() {
return cardCompany;
}
public String getCardType() {
return cardType;
}
}
응답을 받을 객체 생성
class CardResponse {
private List<Card> card;
public List<Card> getCard() {
return card;
}
public void setCard(List<Card> card) {
this.card = card;
}
}
서비스 호출 클래스 생성
public class CardListService {
public static void main(String[] args) {
BarobillApiService barobillApiService = new BarobillApiService();
getCardEx(barobillApiService);
}
public static void getCardEx(BarobillApiService barobillApiService) {
// 입력값 초기화
String certKey = "YOUR_CERT_KEY"; // 연동 인증키 입력
String corpNum = "1234567890"; // 사업자 번호 입력
int availOnly = 1; // 0 : 모든 카드 1 : 사용 가능 카드만 조회
try {
// API 호출
List<Card> cardList = barobillApiService.card.getCardEx(certKey, corpNum, availOnly).getCard();
// 결과 처리
if (cardList == null || cardList.isEmpty()) {
System.out.println("조회된 카드가 없습니다.");
return;
}
if (isErrorCardList(cardList)) {
System.out.println("오류 코드 카드: " + cardList.get(0).getCardNum());
} else {
System.out.println("조회된 카드 목록:");
for (Card card : cardList) {
System.out.println("카드 번호: " + card.getCardNum());
System.out.println("카드사: " + card.getCardCompany());
System.out.println("---------------------------------");
}
}
} catch (Exception e) {
System.err.println("카드 조회 중 오류 발생: " + e.getMessage());
e.printStackTrace();
}
}
// 오류 카드 목록 확인 (예: -12345 패턴)
private static boolean isErrorCardList(List<Card> cardList) {
return cardList.size() == 1 && Pattern.compile("^-[0-9]{5}$").matcher(cardList.get(0).getCardNum()).matches();
}
}
[카드사용내역 API - 카드 사용내역 조회]
바로빌에서 제공하는 카드 사용 내역 조회 API 는 크게 4가지로 나뉜다.
- 원하는 날짜 (단, 200일 사이) 를 지정해서 하는 방법
- 1일씩 조회 하는 것
- 1개월분씩 조회하는 것
- 카드 사용 내역을 조회할 수 있는 URL을 반환
나는 조회 후 결과를 반환하는 세 가지 방법을 구현해보겠다.
먼저 리턴 값을 받을 PagedCardLogEx3 클래스를 생성해 준다.
import java.util.List;
public class PagedCardLogEx3 {
private int currentPage; // 현재 페이지
private int countPerPage; // 페이지 당 건수
private int maxPageNum; // 최대 페이지 수
private int maxIndex; // 전체 건수
private CardLogList cardLogList; // 카드 사용내역 목록
// 기본 생성자
public PagedCardLogEx3() {}
// 필드 전체 초기화 생성자
public PagedCardLogEx3(int currentPage, int countPerPage, int maxPageNum, int maxIndex, CardLogList cardLogList) {
this.currentPage = currentPage;
this.countPerPage = countPerPage;
this.maxPageNum = maxPageNum;
this.maxIndex = maxIndex;
this.cardLogList = cardLogList;
}
// Getter와 Setter
public int getCurrentPage() {
return currentPage;
}
public void setCurrentPage(int currentPage) {
this.currentPage = currentPage;
}
public int getCountPerPage() {
return countPerPage;
}
public void setCountPerPage(int countPerPage) {
this.countPerPage = countPerPage;
}
public int getMaxPageNum() {
return maxPageNum;
}
public void setMaxPageNum(int maxPageNum) {
this.maxPageNum = maxPageNum;
}
public int getMaxIndex() {
return maxIndex;
}
public void setMaxIndex(int maxIndex) {
this.maxIndex = maxIndex;
}
public CardLogList getCardLogList() {
return cardLogList;
}
public void setCardLogList(CardLogList cardLogList) {
this.cardLogList = cardLogList;
}
// 내부 클래스: CardLogList
public static class CardLogList {
private List<CardLogEx3> cardLogEx3;
public CardLogList() {}
public CardLogList(List<CardLogEx3> cardLogEx3) {
this.cardLogEx3 = cardLogEx3;
}
public List<CardLogEx3> getCardLogEx3() {
return cardLogEx3;
}
public void setCardLogEx3(List<CardLogEx3> cardLogEx3) {
this.cardLogEx3 = cardLogEx3;
}
}
}
카드사용내역 API 호출이 비슷하니 공통으로 사용할 서비스 클래스도 만들어 준다.
import java.util.List;
public class CardUseList {
private final BarobillApiService barobillApiService = new BarobillApiService();
// 월 카드사용내역 API 요청
public PagedCardLogEx3 getMonthlyCardLogEx3(String certKey, String corpNum, String id, String cardNum, String baseMonth, int countPerPage, int currentPage, int orderDirection) {
return fetchCardLog("Monthly", certKey, corpNum, id, cardNum, baseMonth, null, countPerPage, currentPage, orderDirection);
}
// 일 카드사용내역 API 요청
public PagedCardLogEx3 getDailyCardLogEx3(String certKey, String corpNum, String id, String cardNum, String baseDate, int countPerPage, int currentPage, int orderDirection) {
return fetchCardLog("Daily", certKey, corpNum, id, cardNum, baseDate, null, countPerPage, currentPage, orderDirection);
}
// 기간 카드사용내역 API 요청
public PagedCardLogEx3 getPeriodCardLogEx3(String certKey, String corpNum, String id, String cardNum, String startDate, String endDate, int countPerPage, int currentPage, int orderDirection) {
return fetchCardLog("Period", certKey, corpNum, id, cardNum, startDate, endDate, countPerPage, currentPage, orderDirection);
}
private PagedCardLogEx3 fetchCardLog(String type, String certKey, String corpNum, String id, String cardNum, String date1, String date2, int countPerPage, int currentPage, int orderDirection) {
PagedCardLogEx3 result = null;
switch (type) {
case "Monthly":
result = barobillApiService.card.getMonthlyCardLogEx3(certKey, corpNum, id, cardNum, date1, countPerPage, currentPage, orderDirection);
break;
case "Daily":
result = barobillApiService.card.getDailyCardLogEx3(certKey, corpNum, id, cardNum, date1, countPerPage, currentPage, orderDirection);
break;
case "Period":
result = barobillApiService.card.getPeriodCardLogEx3(certKey, corpNum, id, cardNum, date1, date2, countPerPage, currentPage, orderDirection);
break;
}
printCardLogResult(result);
return result;
}
private void printCardLogResult(PagedCardLogEx3 result) {
if (result.getCurrentPage() < 0) { // 호출 실패
System.out.println("호출 실패: " + result.getCurrentPage());
} else { // 호출 성공
System.out.printf("페이지: %d / 페이지당 항목 수: %d / 최대 페이지: %d / 최대 항목 수: %d%n",
result.getCurrentPage(), result.getCountPerPage(), result.getMaxPageNum(), result.getMaxIndex());
List<CardLogEx3> logs = result.getCardLogList().getCardLogEx3();
for (CardLogEx3 log : logs) {
System.out.println("카드 번호: " + log.getCardNum());
}
}
}
}
이걸 이제 메인에서 사용해주면 원하는 카드사용내역을 조회할 수 있다.
[마무리]
테스트 코드를 조금 변경 해서 작성해 보았는데 바로빌은 제공하는 정보가 너무 깔끔하고 문서화가 잘 되어 있어서 사용하기 편리하다. 또 원하는 API 서비스만 선택하여 개발한 후 사용한 만큼만 지불하는 시스템이라 가격적인 측면에서도 너무 매력적인 것 같다.
조금 더 살펴보길 원한다면 아래의 링크로 가서 둘러보시는걸 추천드립니당
스크래핑 API 레퍼런스 - 카드조회 API | 바로빌 개발자센터
카드 등록 신청, 카드목록 조회, 카드 사용내역 조회 등 바로빌에서 제공하는 카드조회 API를 사용하는데 필요한 규격과 가이드를 제공합니다.
dev.barobill.co.kr
해당 게시글은 업체로부터 소정의 원고료를 받아 작성되었습니다
'JAVA' 카테고리의 다른 글
[자료구조] Array 와 List 의 차이 (배열과 리스트의 차이) (0) | 2024.11.27 |
---|---|
BufferedReader 와 BufferedWriter (0) | 2024.11.23 |
Java int배열의 오름차순과 내림차순 (0) | 2024.11.21 |
Java int 와 Integer 의 차이 (0) | 2024.11.20 |
Java 문자열 뒤집기 - reverse() (0) | 2024.11.19 |